Appendix
Appendix A - Some useful sprite calls
With all the following calls, user sprites are assumed. This is identified by either +256 or +512 added to the value of R0. Unless otherwise stated you can assume the following.
+256 or +512 R1 is the pointer to the sprite area you set aside
+256 R2 is a pointer to the sprite name
+512 R2 is the sprite actual address
Intialise sprite area
R0 = 9+256
Before making this call you mast set the first two words in your sprite area. These should be !area=0 and area!4=16
Load sprite file
R0 = 10+256
R2 = pointer to file pathname
Save sprite file
R0 = 12+256
R2 = pointer to file pathname
Get sprite from graphics coordinates
R0 = 14+256
R2 = pointer to sprite name
R3 = 0 to exclude palette data, 1 to include it
Returns - R2 = address of sprite
Create empty sprite
R0 = 15+256
R2 = pointer to sprite name
R3 = 0 to exclude palette data, 1 to include it
R4 = width in pixels
R5 = height in pixels
R6 = screen Mode number
Get sprite from user coordinates
R0 = 16+256
R2 = pointer to sprite name
R3 = 0 to exclude palette data, 1 to include it
R4 = left edge OS screen coodinate
R5 = bottom edge OS screen coordinate
R6 = right edge OS screen coordinate
R7 = top edge OS screen coordinate
Returns - R2 = address of sprite
Create mask with all pixels set (solid)
R0 = 29+512
Put sprite to current graphics cursor
R0 = 28+512
R5 = plot action
Plot actions are as below
0 overwrite on screen
1 OR with colour on screen
2 AND with colour on screen
3 EOR with colour on screen
4 invert colour on screen
5 leave colour on screen unchanged
6 AND with colour on screen with NOT of sprite pixel colour
7 OR with colour on screen with NOT of sprite pixel colour
&08 if set, use mask, otherwise don't
&10 ECF pattern 1
&20 ECF pattern 2
&30 ECF pattern 3
&40 ECF pattern 4
&50 giant ECF pattern
Put sprite at user coordinates
R0 = 34+512
R3 = X coordinate
R4 = Y coordinate
R5 = plot action
Read pixel from sprite
R0 = 42+512
R3 = X coordinate
R4 = Y coordinate
Returns - R5 = colour
R6 = tint
Write pixel to sprite
R0 = 42+512
R3 = X coordinate
R4 = Y coordinate
R5 = colour
R6 = tint
Read mask pixels
R0 = 43+512
R3 = X coordinate
R4 = Y coordinate
Return
R5 = status, 0 for transparent, 1 for solid
Write mask pixels
R0 = 44+512
R3 = X coordinate
R4 = Y coordinate
R5 = status
Put scaled sprite
R0 = 52+512
R3 = X coordinate to plot
R4 = Y coordinate to plot
R5 = plot action
R6 = pointer to scale control block
R7 = pointer to pixel translation table, 0 to use sprite colours
The scale control block byte offsets are as below
0 X multiplier
4 Y multiplier
8 X divisor
12 Y divisor
For pixel translation, a sprite pixel colour of N will be plotted as the colour represented by the Nth byte in the table
Switch output to sprite
R0 = 60+512
R2 = sprite address as usual, but if zero will restore screen output
R3 = save area (generally not needed, set to 0)
Switch output to mask
R0 = 61+512
R2 = sprite mask address, 0 for screen output
R3 = save area, normally 0
Appendix B - Screen Modes for standard monitors
Mode Text Graphics Colours Memory
0 80x32 640x256 2 20k
1 40x32 320x256 4 20k
2 20x32 160x256 16 40k
3 80x25 Text only 2 40k
4 40x32 320x256 2 20k
5 20x32 160x256 4 20k
6 40x25 Text only 2 20k
7 40x25 TELETEXT 16 80k
8 80x32 640x256 4 40k
9 40x32 320x256 16 40k
10 20x32 160x256 256 80k
11 80x25 640x250 4 40k
12 80x32 640x256 16 80k
13 40x32 320x256 256 80k
14 80x25 640x250 16 80k
15 80x32 640x256 256 160k
16 132x32 1056x256 16 132k
17 132x25 1056x250 16 132k
Appendix C - VDU variables (sub-set)
Number Meaning
1 Maximum column number for printing
2 Maximum row number for printing
4 Barrel shift right to convert X coordinate to screen pixels
5 Barrel shift right to convert Y coordinate to screen pixels
6 Screen line length in bytes
7 Size in bytes of the visible screen
148 Address of screen start use by VDU drivers
149 Address of displayed screen start
150 Total number of bytes currently available to screen
Appendix D - VDU commands
Code Ctrl Bytes Meaning
0 @ 0 Does nothing
1 A 1 Sends next character to printer only
2 B 0 Enables printer
3 C 0 Disables printer
4 D 0 Writes characters at text cursor
5 E 0 Writes characters at graphics cursor
6 F 0 Enables VDO driver
7 G 0 Generates bell sound
8 H 0 Moves cursor back one character or deletes previous character
9 I 0 Moves cursor on one space
10 J 0 Moves cursor down one line
11 K 0 Moves cursor up one line
12 L 0 Clears text area
13 M 0 Moves cursor to start of current line
14 N 0 Enables page mode
15 O 0 Disables page mode
16 P 0 Clears graphics area
17 Q 1 Defines text colour
18 R 2 Defines graphics colour
19 S 0 Defines logical colour
20 T 0 Restores default colours
21 U 0 Disables VDU driver or deletes current line
22 V 1 Selects screen mode
23 W 9 General purpose command
24 X 8 Defines graphics window
25 Y 5 PLOT
26 Z 0 Restores default windows
27 [ 0 Does nothing
28 \ 4 Defines text window
29 ] 4 Defines graphics origin
30 ^ 0 Homes text cursor
31 _ 2 Moves text cursor
32-255 Display characters
Appendix E - Plot Codes
Plot codes are in groups of eight. Below are the base number of each group.
Dec Hex Meaning
0 00 Solid line including both end points
8 08 Solid line excluding final point
16 10 Dotted line including both end points
24 18 Dotted line excluding final point
32 20 Solid line excluding first point
40 28 Solid line excluding both end points
48 30 Dotted line excluding first point
56 38 Dotted line excluding both end points
64 40 Point plot
72 48 Horizontal line fill to non-background (left and right)
80 50 Triangle fill
88 58 Horizontal line fill to background (right only)
96 60 Rectangle fill
104 68 Horizontal line fill to foreground (left and right)
112 70 Parallelogram fill
120 78 Horizontal line fill to non-foreground (right only)
128 80 Flood fill to background
136 88 Flood fill to foreground
144 90 Circle outline
152 98 Circle fill
160 A0 Circular arc
168 A8 Segment
176 B0 Sector
184 B8 Block copy/move
192 C0 Ellipse outline
200 C8 Ellipse fill
Within eack block the offset from the base number has the following meaning.
0 Move cursor relative to last point visited
1 Draw relative, using current foreground colour
2 Draw relative, using logical inverse colour
3 Draw relative, using current background colour
4 Move cursor to absolute coordinates
5 Draw absolute, using current foreground colour
6 Draw absolute, using logical inverse colour
7 Draw absulute, using current background colour
Block copy and move are exceptions whose codes are
0 Move only, relative
1 Move rectangle relative
2 Copy rectangle relative
3 Copy rectangle relative
4 Move only, absolute
5 Move rectangle absolute
6 Copy rectangle absolute
7 Copy rectangle absolute
Appendix F - Negative inkey vaues
Key Number Key Number
Cursor up -58 Mouse select -10
Cursor down -42 Mouse menu -11
Cursor left -26 Mouse adjust -12
Cursor right -122 = -94
Print -33 ' -103
F1 -114 - -24
F2 -115 . -104
F3 -116 / -105
F4 -21 [ -57
F5 -117 \ -121
F6 -118 ] -89
F7 -23 ; -88
F8 -119 Esc -113
F9 -120 Tab -97
F10 -31 Caps Lock -65
F11 -29 Scroll Lock -32
F12 -30 Num Lock -78
A -66 Break -47
B -101 ~\` -46
C -83 Currency -47
D -51 Back Space -48
E -35 Insert -62
F -68 Home -63
G -84 Page Up -64
H -85 Page Down -79
I -38 '\" -80
J -70 Shift (either/both) -1
K -71 Alt (either/both) -3
L -87 Shift (left/right) -4/-7
M -102 Ctrl (left/right) -5/-8
N -86 Alt (left/right -6/-9
O -55 Space bar -99
P -56 Delete -90
Q -17 Return -74
R -52 Copy -106
S -82 Keypad 0 -107
T -36 Keypad 1 -108
U -54 Keypad 2 -125
V -100 Keypad 3 -109
W -34 Keypad 4 -123
X -67 Keypad 5 -124
Y -69 Keypad 6 -27
Z -98 Keypad 7 -28
0 -40 Keypad 8 -48
1 -49 Keypad 9 -44
2 -50 Keypad + -59
3 -18 Keypad - -60
4 -19 Keypad . -77
5 -20 Keypad / -75
6 -53 Keypad # -91
7 -37 Keypad * -92
8 -22 Keypad Enter -61
9 -39
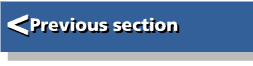 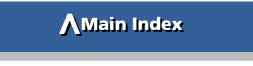
|